An Items bought with widget. Introduced in v2.16.
The ItemsBoughtWith_Listing widget is designed to be used within an Item Detail page. By default, the widget will display up to the top 10 most popular Items purchased by other Shoppers at the same time as the Item being viewed. The listing will display the Item's photo, name, stock code and Web Price (when to the Shopper).
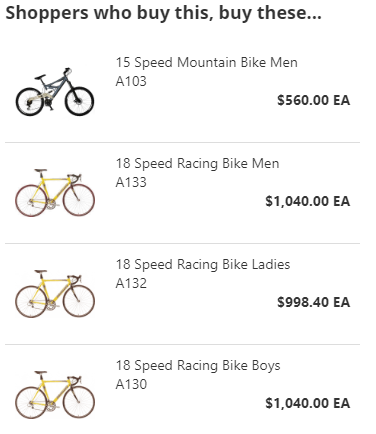
Widget options provide a way to customize widgets in various ways. Widget options are sent to the widget when loaded with the LoadWidgetControl.
To override the default maximum number of Items to show in the widget, set the maxItemsToShow option to any integer greater than 0. The default is 10.
To specify a specific height for the widget and force scrolling when the number of results would require additional space, set the widgetHeight option to either a percentage or number of pixels as an integer. The default is '100%'.
The default headline text displayed above the listing is 'Shoppers who buy this, buy these...' but the text can be changed by setting the value of the headlineText option.
When determining the Item for which the widget compares order history to, the stock code of the item is determined from the Item Detail page address URL, however this can be overridden using the stockCode option.
Item Photo Options
The width in pixels of the Item photo can be set using the photoWidth option. The default when not set is 80. The height of the photo will then scale in proportion to the width automatically.
Image alternate text can be set using the photoAltText option. The default is the stock code of the Item followed by a hyphen (-) and then the name of the Item (e.g. 'A101 - 15 Speed Mountain Bike').
While the Item photo is loading an image placeholder will load, and if no photo is found this image will remain visible. To set the image to use, set the value of the pictureNotFoundImageUrl option. The default is '/ecommerce/images/photo-not-found.png'.
CSS Class Options
The default CSS Class used for the photo's <img> tag is 'ListingPhoto', but it can be customized using the photoCssClass option.
The containing <div> tag that holds the Item detail text, has a default CSS Class of 'ListingItemDetails', and can be customized using the detailsCssClass option.
The priceCssClass option can be used for customizing the CSS Class of the <span> that contains the price of the Item. The default class is 'WebPrice'.
All ID attributes for elements used in the ItemsBoughtWith_Listing widget end with a suffix of '_ItemsBoughtWith' and for those elements within the repeating HTML for each Item in the listing also will have the Stock Code of the base Item contained within so as to provide a predictable format to the element IDs.
For example:
- The <div> that serves as the wrapper for the widget has an element ID of 'divWrapper_ItemsBoughtWith'.
- The <img> for Item A103 in the listing has an element ID of 'imgItemPhoto_ItemsBoughtWith_A103'.
See an example of how to load and configure this widget in SitePages.config.
<!-- Example 1: Options set with default values.
Item determined by evaluating URL of the Item Detail Page in page address
e.g https://www.yourdomain.com/ecommerce/product/A100/15-Speed-Mountain-Bike-Boys
-->
<Control src="LoadWidgetControl.ascx" Name="BoughtWith Widget"
FileLocation="ItemsBoughtWith_Listing.html"
Options="
maxItemsToShow: 10,
headlineText: 'Shoppers who buy this, buy these...',
photoWidth: 80,
widgetHeight: '100%',
pictureNotFoundImageUrl: '/ecommerce/images/photo-not-found.png',
photoCssClass: 'ListingPhoto',
detailsCssClass: 'ListingItemDetails',
priceCssClass: 'WebPrice'"
/>
<!-- Example 2: Use all default option values except:
stockCode - Item forced to override anything retreived from the URL.
maxItemToShow - show only top 5.
photoWidth - Set to custom of 72 pixels.
pictureNotFoundImageUrl - use a custom image in the theme.
e.g https://www.yourdomain.com/ecommerce/product/A100/15-Speed-Mountain-Bike-Boys
-->
<Control src="LoadWidgetControl.ascx" Name="BoughtWith Widget"
FileLocation="ItemsBoughtWith_Listing.html"
Options="
stockCode:'A100'
maxItemsToShow: 5,
photoWidth: 72,
widgetHeight: '100%',
pictureNotFoundImageUrl: '/ecommerce/site/themes/images/myphoto-not-found.png'"
/>
The following is the source code for this widget.
Developer's Note:
To create a custom version of the widget, copy all of the code below into a file of the same name and place it into your Site's widgets folder (e.g., ../YourSiteFolder/Widgets). The CyberStore page engine will then override the default source with your customized version.
<div id="divWrapper_ItemsBoughtWith">
<h4 id="h4Headline_ItemsBoughtWith"></h4>
<div class="list-container_ItemsBoughtWith">
<div id="listWidget_ItemsBoughtWith"></div>
</div>
<script type="text/html" id="tmplItems_ItemsBoughtWith">
<div class="product">
<img id="imgItemPhoto_ItemsBoughtWith_<%= StockCode %>" src="<%= widgetOptions.pictureNotFoundImageUrl || (SITE_PATHS.application + '/images/photo-not-found.png') %>"
width="80" class="ListingPhoto" alt="<%= StockCode %> - <%= Name %>" />
<div id="divItemDetails_ItemsBoughtWith_<%= StockCode %>" class="ListingItemDetails">
<%= Name %><br />
<%= StockCode %>
</div>
<div id="divPrice_ItemsBoughtWith_<%= StockCode %>" >
<span id="spnPrice_ItemsBoughtWith_<%= StockCode %>" class="WebPrice"></span>
</div>
</div>
<script>
if (widgetOptions.photoCssClass != null) { SetAttribute(widgetOptions.photoCssClass, 'class', 'imgItemPhoto_ItemsBoughtWith_<%= StockCode %>'); }
if (widgetOptions.priceCssClass != null) { SetAttribute(widgetOptions.priceCssClass, 'class', 'spnPrice_ItemsBoughtWith_<%= StockCode %>'); }
if (widgetOptions.detailsCssClass != null) { SetAttribute(widgetOptions.detailsCssClass, 'class', 'divItemDetails_ItemsBoughtWith_<%= StockCode %>'); }
if (widgetOptions.photoWidth != null) { SetAttribute(widgetOptions.photoWidth, 'width', 'imgItemPhoto_ItemsBoughtWith_<%= StockCode %>'); }
if (widgetOptions.photoAltText != null) { SetAttribute(widgetOptions.photoAltText, 'alt', 'imgItemPhoto_ItemsBoughtWith_<%= StockCode %>'); }
MakeAJAXCall('Inventory.GetItemPhoto', { StockCode: '<=StockCode>' }, SetAttribute, { attribute: 'src', element: 'imgItemPhoto_ItemsBoughtWith_<%= StockCode %>', setBlank:false });
MakeAJAXCall('Price.GetDisplayPriceByStockCode', { StockCode: '<=StockCode>', Quantity: 1, UOM: 0 }, SetWebPrice, 'spnPrice_ItemsBoughtWith_<%= StockCode %>');
</script>
</script>
</div>
<script type="text/javascript">
$(function () {
var Params = {
StockCode: widgetOptions.stockCode || GetStockCodeFromPage(),
HowMany: widgetOptions.maxItemsToShow || 10
};
MakeAJAXCall('Inventory.GetTopItemsBoughtWithStockCodeByOthersToBuy', Params, bindItemsBoughtWith);
});
function bindItemsBoughtWith(d) {
var i = JSON.parse(d);
if (Object.keys(i).length > 0) {
$('#h4Headline_ItemsBoughtWith').text(widgetOptions.headlineText || 'Shoppers who buy this, buy these...');
$("#listWidget_ItemsBoughtWith").dxList({
dataSource: i,
height: widgetOptions.widgetHeight || '100%',
itemTemplate: $("#tmplItems_ItemsBoughtWith"),
onItemClick: (e) => {
document.location = SITE_PATHS.application + '/product/' + e.itemData.StockCode;
}
});
}
}
</script>